AS3 Game Object Editor How To
To expose a class for runtime editing, simply add the Editable
metadata to your variables and accessors (See Metadata syntax and Class markup).
After that's done, you need to register the class with the Editor
class. Somewhere in your code, before you create your objects, create a new Editor
class and register whichever classes you want with it:
this.m_editor = new Editor( this.stage );
// Ball is a class that's had Editable metadata added to it
this.m_editor.registerClass( Ball );
// this will add the Editor to the stage
this.m_editor.visible = true;
This will perform everything necessary in the background to extract the editable variables from your class. From this moment on, whenever a class of the same type registered is created and added to the Stage
(or object on the Stage
), that object gets added into the list of objects that we can edit.
Explicitly adding objects / blitting frameworks
If you want to add a non-DisplayObject
with the Editor, then you'll need to explicitly register it (you can explicitly register DisplayObjects
as well, but they're added automatically once they're on the stage). Keep this in mind if you're using something like Flixel, where all the graphics are blitted, so your classes aren't getting added to the stage, per-se.
Call registerObject()
and pass in your object to add it to the list of objects to edit.
Explicitly removing objects
Objects that are added to the Editor that are also DisplayObject
s are removed automatically once they're removed from the stage. For non-DisplayObject
s or if you want to control when it's removed, you can call unregisterObject()
and pass in the object you want to remove.
Showing/hiding the Editor
Showing/hiding the Editor
is done through its visible
property (it's not visible by default). When visible, it's added to the Stage
automatically. When it's shown, you can access the list of editable objects through a drop down list. There's also an option to click on a DisplayObject to set it as the current editable object. If you're having trouble figuring out which object is the current, there's also an option to highlight the currently selected object.
When you select an object, all of the editable variables that have been found are shown with their various components. Go forth and edit!
Auto-update non-watch type vars
By default, watch
vars are the only Editable types that are updated every frame. If you need to update all variable types (e.g. input
, slider
etc), then simply set the updateOnlyWatchVars
bool on the Editor
to false
.
What have I done?
While editing your objects, you have the ability to save the edited variables in XML
form for either the currently active object, or all objects that we're currently tracking. XML
data is also copied to the clipboard if file saving is disabled for some reason.
When saved, the data for an object is available in a node with the same name as the object. For example, if you had two objects, one unnamed (will appear by default as something like "instance100"
) and the other named "second"
, your saved XML
data would be in the form of:
<game>
<instance100>
...
</instance100>
<second>
...
</second>
</game>
Troubleshooting
If your editing data suddenly disappears, or if you want to allow editing during a release build, make sure and add
-keep-as3-metadata+=Editable
to the project settings (Project > Properties > Compiler Options > Additional Compiler Options)
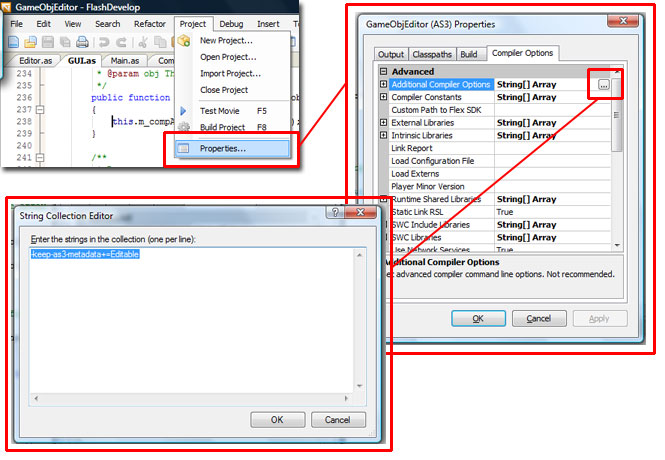
minimalcomps
This project uses minimalcomps (v0.9.9) from Bit101: http://code.google.com/p/minimalcomps/ or https://github.com/minimalcomps.
If you download the SWC, you'll need to have minimal comps in your project. If you download the ZIP, you'll find the minimalcomps SWC in the libs folder.
Comments
Submit a comment